I had earlier written how we could create Animated Navigation Menu using CSS3. Now, in this post I am going to show how we can create stack of papers effect using CSS3 and then how we can add animations and ribbons using CSS only.
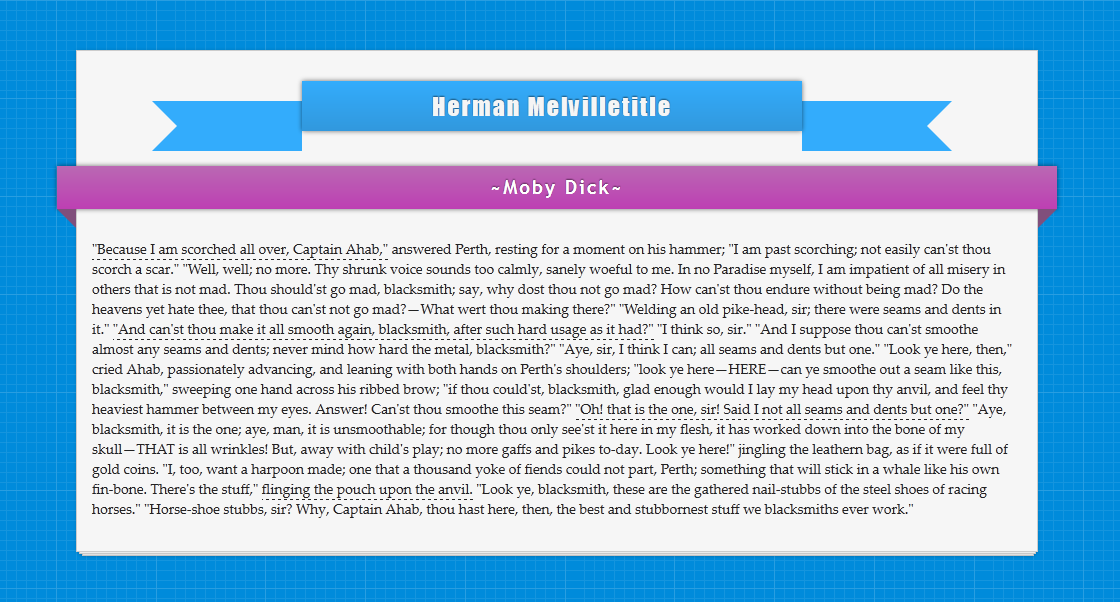
Live Demo // Download
Basic Stack of Papers
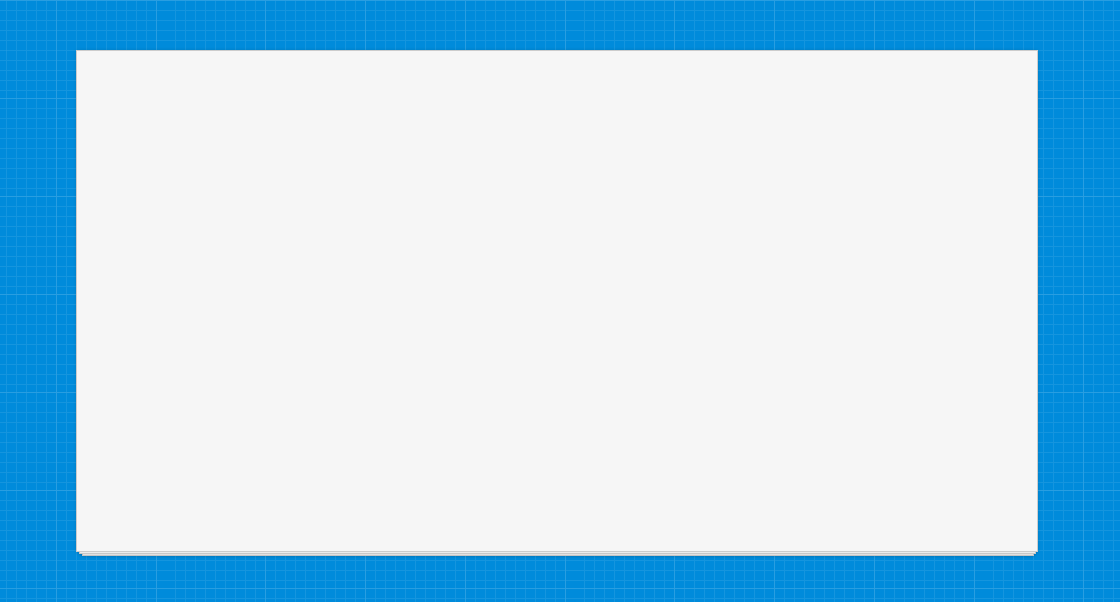
Setting up basic stack of papers is easy, the code for it is below and you can see a live demo here.
The CSS
{code type=CSS}
body {
background: #333 url(http://priteshgupta.com/demos/papers/bg.png);
}
.stack {
background: #f6f6f6;
border: 1px solid #ccc;
height: 500px;
margin: 50px auto;
position: relative;
width: 960px;
-webkit-box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
-moz-box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
}
.stack:after, .stack:before {
background: #f6f6f6;
border: 1px solid #ccc;
bottom: -3px;
content: ”;
height: 500px;
left: 2px;
position: absolute;
width: 955px;
z-index: -10;
-webkit-box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
-moz-box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
}
.stack:before {
bottom: -5px;
left: 5px;
width: 950px;
}
{/code}
The HTML
{code type=HTML}
{/code}
However, if you will like more than three stack of papers, use the below CSS and see a live demo here.
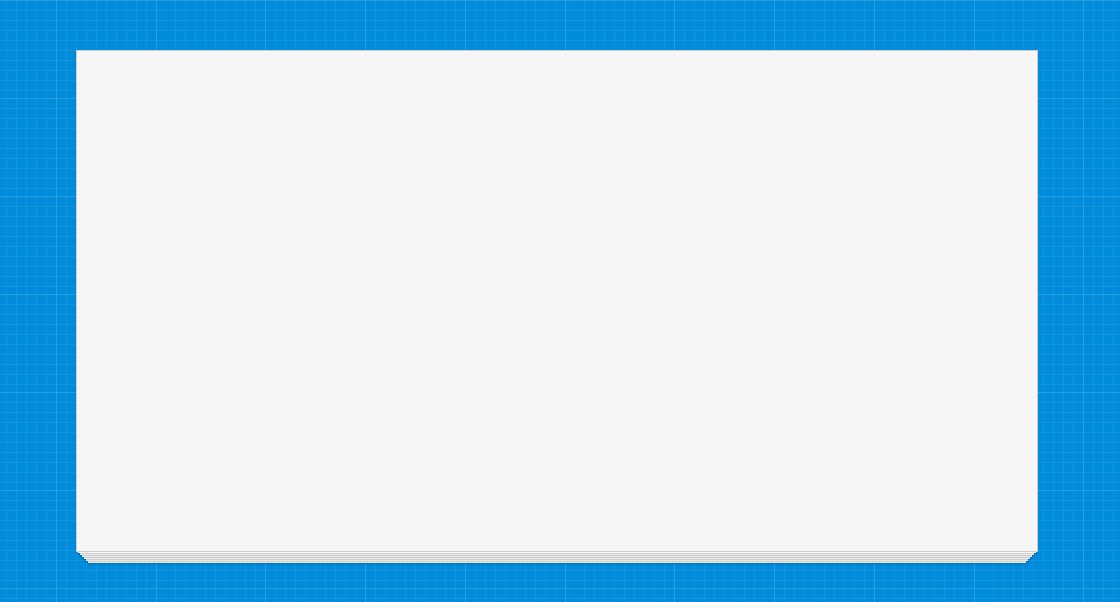
{code type=CSS}
body {
background: #333 url(http://priteshgupta.com/demos/papers/bg.png);
}
.stack {
background: #f6f6f6;
border: 1px solid #ccc;
height: 500px;
margin: 50px auto;
width: 960px;
-webkit-box-shadow: 0 0 0 hsla(0, 0%, 0%, .5), 0 3px 0 -2px #f6f6f6, 0 3px 2px -2px hsla(0, 0%, 0%, .4), 0 7px 0 -4px #f6f6f6, 0 7px 2px -4px hsla(0, 0%, 0%, .4), 0 11px 0 -6px #f6f6f6, 0 11px 2px -6px hsla(0, 0%, 0%, .4), 0 15px 0 -8px #f6f6f6, 0 15px 2px -8px hsla(0, 0%, 0%, .4), 0 19px 0 -10px #f6f6f6, 0 19px 2px -10px hsla(0, 0%, 0%, .4), 0 23px 0 -12px #f6f6f6, 0 23px 2px -12px hsla(0, 0%, 0%, .4);
-moz-box-shadow: 0 0 0 hsla(0, 0%, 0%, .5), 0 3px 0 -2px #f6f6f6, 0 3px 2px -2px hsla(0, 0%, 0%, .4), 0 7px 0 -4px #f6f6f6, 0 7px 2px -4px hsla(0, 0%, 0%, .4), 0 11px 0 -6px #f6f6f6, 0 11px 2px -6px hsla(0, 0%, 0%, .4), 0 15px 0 -8px #f6f6f6, 0 15px 2px -8px hsla(0, 0%, 0%, .4), 0 19px 0 -10px #f6f6f6, 0 19px 2px -10px hsla(0, 0%, 0%, .4), 0 23px 0 -12px #f6f6f6, 0 23px 2px -12px hsla(0, 0%, 0%, .4);
box-shadow: 0 0 0 hsla(0, 0%, 0%, .5), 0 3px 0 -2px #f6f6f6, 0 3px 2px -2px hsla(0, 0%, 0%, .4), 0 7px 0 -4px #f6f6f6, 0 7px 2px -4px hsla(0, 0%, 0%, .4), 0 11px 0 -6px #f6f6f6, 0 11px 2px -6px hsla(0, 0%, 0%, .4), 0 15px 0 -8px #f6f6f6, 0 15px 2px -8px hsla(0, 0%, 0%, .4), 0 19px 0 -10px #f6f6f6, 0 19px 2px -10px hsla(0, 0%, 0%, .4), 0 23px 0 -12px #f6f6f6, 0 23px 2px -12px hsla(0, 0%, 0%, .4);
}
{/code}
Animating Stack of Papers
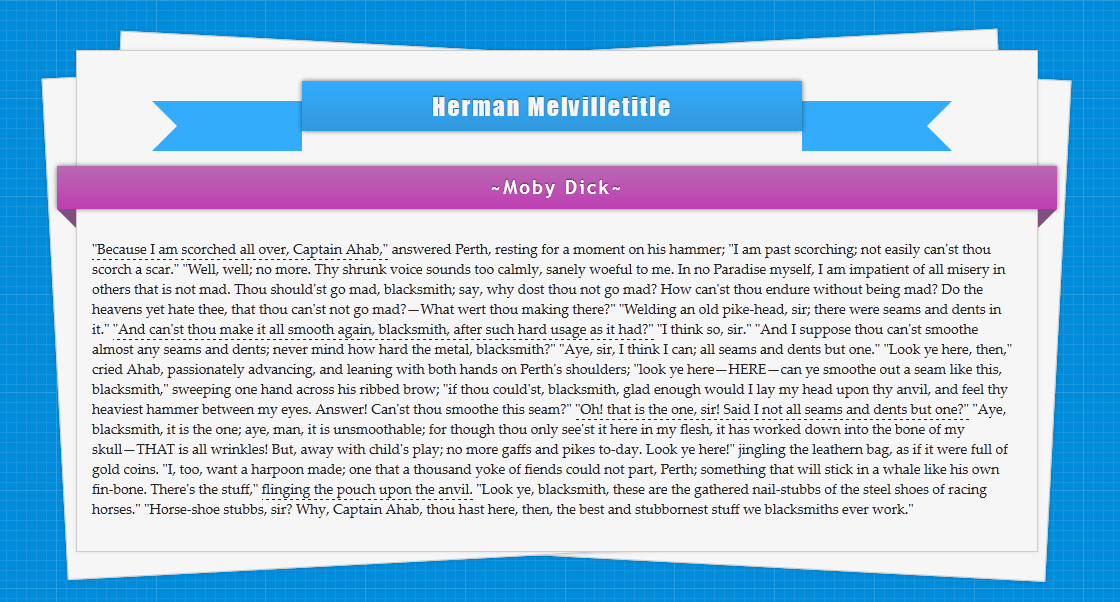
Now, I have added some content in it along with two CSS Ribbons and a simple animation to spread the stack of papers, though the easing animation is only available in Firefox(Since it only supports transition tags with Pseudo-elements). This is the final version, you can see a live demo here.
The CSS
{code type=CSS}
body {
background: #333 url(http://priteshgupta.com/demos/papers/bg.png);
font-family: ‘Palatino Linotype’, ‘Book Antiqua’, Palatino, serif;
}
p {
margin: 15px;
font-size: 14px;
font-family: ‘Palatino Linotype’, ‘Book Antiqua’, Palatino, serif;
color: #222;
position: relative;
float: left;
}
.stack {
background: #f6f6f6;
border: 1px solid #ccc;
height: 500px;
margin: 50px auto;
position: relative;
width: 960px;
-webkit-box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
-moz-box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
box-shadow: 0 0 3px hsla(0, 0%, 0%, .1);
}
.stack:after, .stack:before {
background: #f6f6f6;
border: 1px solid #ccc;
bottom: -3px;
content: ”;
height: 500px;
left: 2px;
position: absolute;
width: 955px;
z-index: -10;
-webkit-box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
-moz-box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
box-shadow: 0 0 3px hsla(0, 0%, 0%, .2);
-webkit-transition: all .5s ease-out;
-moz-transition: all .5s ease-out;
-o-transition: all .5s ease-out;
}
.stack:before {
bottom: -5px;
left: 5px;
width: 950px;
}
.stack:hover:after {
-webkit-transform: rotate(-3deg) translate(-25px, 0);
-moz-transform: rotate(-3deg) translate(-25px, 0);
-ms-transform: rotate(-3deg) translate(-25px, 0);
-o-transform: rotate(-3deg) translate(-25px, 0);
transform: rotate(-3deg) translate(-25px, 0);
-webkit-transition: all .5s ease-out;
-moz-transition: all .5s ease-out;
-o-transition: all .5s ease-out;
}
.stack:hover:before {
-webkit-transform: rotate(3deg) translate(25px, 0);
-moz-transform: rotate(3deg) translate(25px, 0);
-ms-transform: rotate(3deg) translate(25px, 0);
-o-transform: rotate(3deg) translate(25px, 0);
transform: rotate(3deg) translate(25px, 0);
-webkit-transition: all .5s ease-out;
-moz-transition: all .5s ease-out;
-o-transition: all .5s ease-out;
}
#main {
font-family: Impact, Charcoal, sans-serif;
letter-spacing: 2px;
margin-left:75px;
margin-top: 50px;
text-shadow: 0 -1px 1px hsla(0, 0%, 0%, .5);
}
#menu {
background: #33acfc;
width: 500px;
height: 50px;
color: #F5F5F5;
font-size: 24px;
text-align: center;
position: relative;
top: -20px;
background-image: -webkit-gradient(linear, left top, left bottom, from(#33acfc), to(#3198dd));
background-image: -moz-linear-gradient(top, #33acfc, #3198dd);
background-image: -o-linear-gradient(top, #33acfc, #3198dd);
-moz-box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
-webkit-box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
}
#menu span {
position: relative;
top: 10px;
}
.cont {
background: #33acfc;
width: 100px;
height: 50px;
}
.edge-right {
border-color: #33acfc transparent #33acfc #33acfc;
border-style:solid;
border-width:25px;
width: 0;
height: 0;
position: relative;
}
.edge-left {
border-color: #33acfc #33acfc #33acfc transparent;
border-style:solid;
border-width:25px;
width: 0;
height: 0;
position: relative;
}
.left {
float: left;
}
.right {
float: right;
}
.ribbon {
width: 980px;
font-size: 19px;
font-family: ‘Trebuchet MS’, Helvetica, sans-serif;
letter-spacing: 2px;
text-shadow: 0 -1px 1px hsla(0, 0%, 0%, .5);
position: relative;
background: #ba89b6;
color:#FFF;
text-align: center;
padding: 10px;
margin-left: -20px;
-moz-box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
-webkit-box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
box-shadow: 0px 0px 4px rgba(0, 0, 0, 0.55);
background-image: -webkit-gradient(linear, left top, left bottom, from(#ba68b3), to(#bd3fb3));
background-image: -moz-linear-gradient(top, #ba68b3, #bd3fb3);
background-image: -o-linear-gradient(top, #ba68b3, #bd3fb3);
}
.ribbon .ribbon-content:before, .ribbon .ribbon-content:after {
content: “”;
position: absolute;
display: block;
border-style: solid;
border-color: #804f7c transparent transparent transparent;
bottom: -1em;
}
.ribbon .ribbon-content:before {
left: 0;
border-width: 1em 0 0 1em;
}
.ribbon .ribbon-content:after {
right: 0;
border-width: 1em 1em 0 0;
}
a {
text-decoration:none;
color: inherit;
border-bottom: dashed 1px #222;
-webkit-transition: all 1s ease-out;
-moz-transition: all 1s ease-out;
-o-transition: all 1s ease-out;
}
a:hover {
background:rgba(51, 172, 252, 0.35);
-webkit-transition: all 1s ease-out;
-moz-transition: all 1s ease-out;
-o-transition: all 1s ease-out;
}
{/code}